REST-assured and Salesforce Quick Start
- Loupe Testware
- Mar 16, 2021
- 3 min read
Salesforce provides RESTful API's that allow us to interact with all the objects with your organisation, whether they are standard or custom made.
From a testing perspective this is awesome, as they provide a "testable interface" at the integration level of the stack. This of course allow's us to create automated tests that are quick to write, easier to maintain, faster to run and easy to slot into your CI/CD pipeline!
We've done a couple of Salesforce projects and Postman was our go to tool of choice. Whilst we love Postman, we also love to treat our automated tests like any other development project, putting it under source control and managing it accordingly. As Postman collections are one bit json file, its tricker to track specific changes using Git, than say using a Rest-assured project.
So we decided to get Rest-assured working against our Salesforce Developer environment, and share a quick guide that should help you get started with interacting with the Salesforce API's, using Rest-assured.
Authentication Details
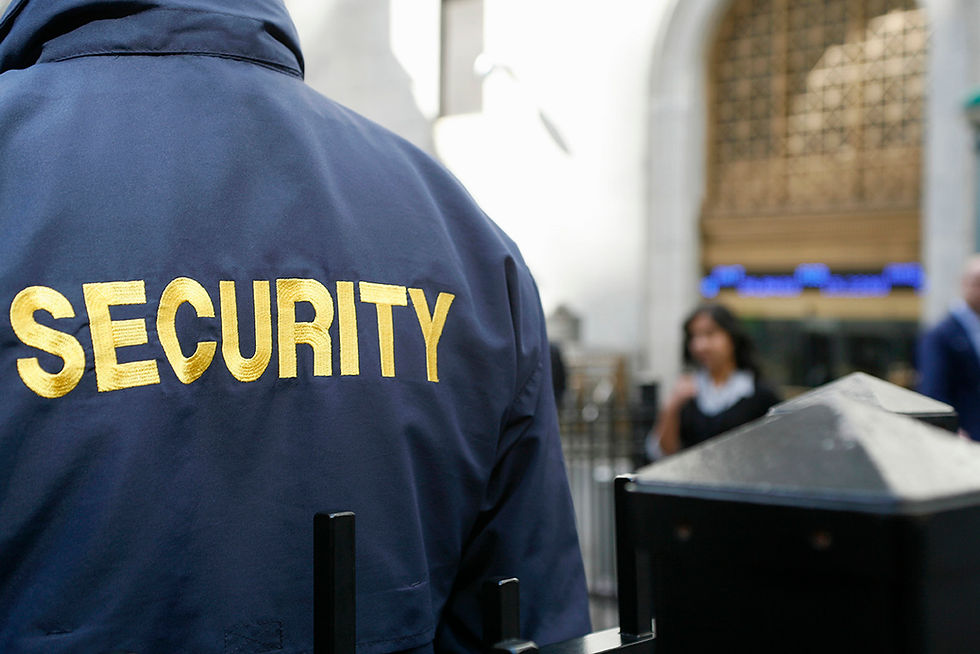
The first (and maybe biggest) challenge to overcome with interacting with the Salesforce API's is authentication.
Before you can connect to your Salesforce Org with an external application, you'll need to create a Connected App.
Once you've created your connected app, grab the client id (Consumer Key) and client secret (Consumer Secret), you'll need those later.
Next you'll need to Reset your Security Token, again you'll need the new token that lands in your email box later.
Now you have all the information you need to post a request to the Salesforce Token endpoint, and get a valid token that you can use in subsequent requests.
Build your requests
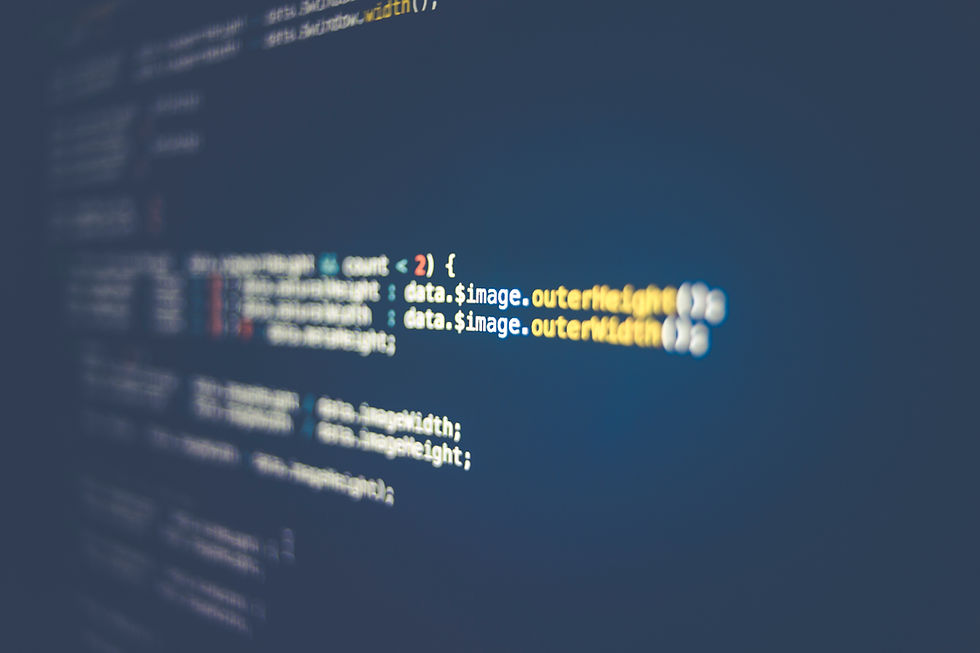
The code snippet below shows an example post request, using Rest-assured, the Salesforce Token endpoint, using all the details we captured in the previous steps. In the example below, we have have extracted the "access_token" and returning the value so we can use it in a subsequent request.
Note - the url you post to may be either login.salesforce... or test.salesforce.... depending on the environment under test.
Get the Auth Token
private String nameOfYourFunction() { return given().urlEncodingEnabled(true) .param("username", "yourSalesforceUsername") .param("password", "yourSalesforcePassword" + "yourSecurityToken") .param("client_id", "yourConsumerKey") .param("client_secret", "yourConsumerSecret") .param("grant_type", "password") .header("Accept", "application/json") .header("Content-Type", "application/x-www-form-urlencoded") .when(). post("https://login.salesforce.com/services/oauth2/token"). then(). assertThat().statusCode(200).log().body().extract().path("access_token"); }
Create a new Account Object
Now that we have an auth token to work with, we can go ahead and post to one of the many Salesforce API's that allow us to interact with the Salesforce Objects, In the example below we have used ReadyAPI to create a new account object. The example includes the minimum amount of data needed working with a totally "vanilla" Account object - a name. You can see that after the assertion we extract the id of the new Account Object created, so we can pass that value into subsequent requests to patch or delete if we want.
@Test public void createAnAccountIn SalesforceUsingTheAPI() { Account account = new Account(); account.setName("Loupe Testware"); given(). contentType(ContentType.JSON). header("Authorization", "Bearer " + nameOfYourFunction()). body(account). log().body(). when(). post("urlForYourSalesForceInstance/services/data/v51.0/sobjects/Account"). then(). assertThat(). statusCode(201). log().body(). extract().path("id"); }
Extend your "framework"
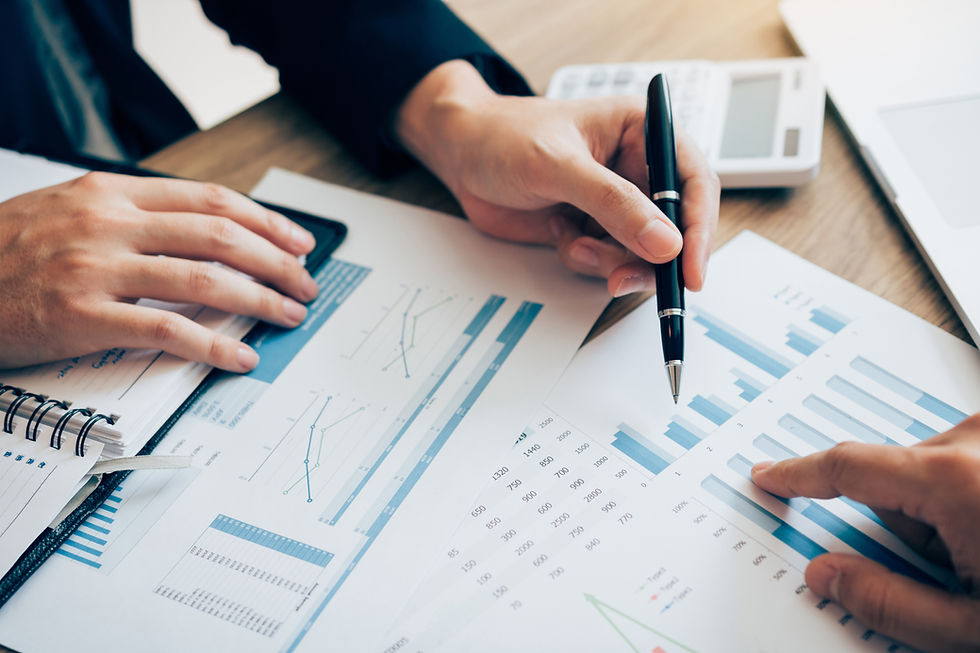
Once you have done the hard work of sorting out authentication and figuring out how to post, get, delete etc.. to each endpoint, your then into the realms of extending your framework to include any reporting, building up a library of Java objects to represent JSON bodies that you wan to to post (a but like a POM for UI frameworks) and integrating into CI.
Thank you for reading, we hope you found this short quick start helpful. If you need any more information or assistance on this topic we are more than happy to help! You can contact us at info@loupetestware.com, or by filling out one of the contact us forms on this site.
Comments